Introduction
The Internet of Things (IoT) represents a complex ecosystem of interconnected devices to collect, process, and transmit the data to other devices or systems. However, beneath the surface of this impressive technology, there are many challenges that developers must navigate. One of the primary obstacles is the inherent complexity and interoperability issues that arise when multiple devices with varying protocols and architectures attempt to communicate. The seamless integration of these diverse systems is critical, yet achieving it is no small feat.
As IoT systems spread across industries, the task of ensuring the seamless data exchange between different devices becomes increasingly daunting. Developers work hard to connect many devices together smoothly so they can share data well. This is compounded by the varying capabilities of these devices—some are equipped with advanced sensors and high computational power, while others might be simpler, low-energy nodes.
In this blog post, we discuss the complexity of collecting and transmitting data from actual devices (Fitbit smartwatch) to their counterparts. We'll explore how data flows from real-world devices to other devices or platforms, where this information can be processed and acted upon. Importantly, we highlight a critical caveat in IoT development: what works in a simulator may not always translate effectively to real-world applications. Furthermore, we discuss a significant challenge in the development process where some devices lack dedicated simulators, presenting unique challenges for developers who must test different functionality during the development process in the real devices.
Through this discussion, we aim to shed light on the strategies that worked for us and the challenges that need attention from both researchers and device manufacturers.
Transfer Data from Fitbit Smartwatch to other Layers of IoT System
Simulator Approach
The company provides a device and phone simulator that allows you to build applications and clock faces for Fitbit OS without needing to purchase a device. This setup is ideal for developers to become familiar with Fitbit functionalities.
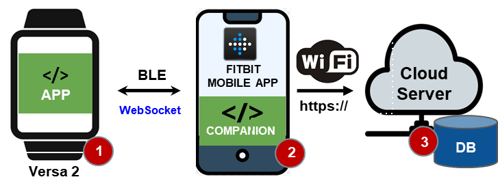
In the above architecture, the fitbit app is responsible for sending the data and transfering this data to companion. Below listing is an example to capture the heart-rate data from the fitbit watch.
// HEART RATE SENSOR CODE
if (HeartRateSensor) {
console.log("This device has a HeartRateSensor!");
const heartRateSensor = new HeartRateSensor();
heartRateSensor.addEventListener("reading", () => {
console.log(`HEART RATE: ${heartRateSensor.heartRate}`);
var heartRate = { heartRate: heartRateSensor.heartRate };
sendMessageToCompanion(heartRate);
});
heartRateSensor.start();
} else {
console.log("This device does NOT have a HeartRateSensor!");
}
Once collected, data is sent to companion by invoking the below segment of code.
function sendMessageToCompanion(message) {
if (peerSocket.readyState === peerSocket.OPEN) {
console.log("Sending message to companion: " + JSON.stringify(message));
peerSocket.send(JSON.stringify(message));
} else {
console.log("Error while sending message: Connection is not open");
}
}
Real Device Approach
Once tested in simulators, the application must be deployed on a real device. However, what works in a simulator may not work on real devices. In our experiments, we found that this approach can succeed on real devices with minor modifications to the 'package.json' file. The key changes includes: Permissions and buildTargets.
{
"requestedPermissions": [
"access_activity",
"access_user_profile",
"access_heart_rate",
"access_location",
"access_internet",
"run_background",
"access_exercise",
"access_calendar",
"access_sleep"
],
"buildTargets": [
"mira"
]
}
However, the cloud server must be secured (https). The above option does not support self-signed certificates. To overcome this challenge, we can add another layer with mobile application server that runs in the same device with fitbit app. This will enables local communication between the two apps. The server app receives the data from companion through fitbit mobile app and then can transfer this data either to the cloud or somewhere else. This scenario is illustrated in Figure 2.
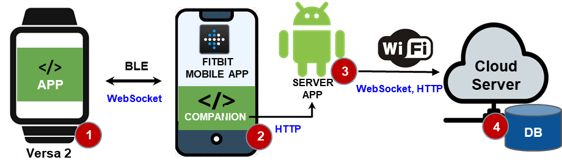
Fitbit to Buddy Robot Interaction
We can create an interesting scenario where a Fitbit watch can trigger some actions that can be executed by the Buddy Robot.
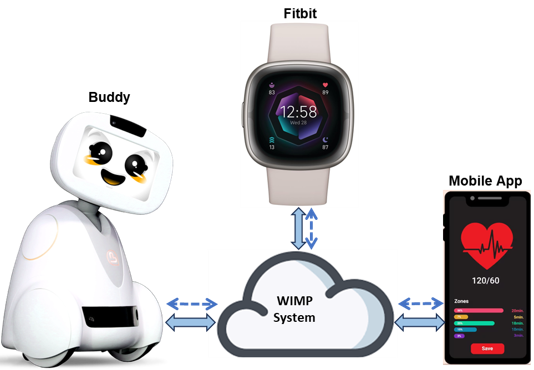
Although such a scenario looks interesting to many developers, the problem remains in how such a system can be tested.
Summary of Challenges & Recommendations
During our experimentation, we realized that the following key challenges for developing IoT systems:
Interoperability Between Devices. For the devices to communicate seamlessly, developers need to be familiar with different communication protocols and standards among diverse IoT devices to ensure they can connect and interact effectively. However, to be familiar with all communication protocols and technologies used in IoT system may not be realistic for one developer. The possible solution to minimize this is to have APIs for each device to help developers interact with those devices by just calling those APIs.
Relying on Simulators. Using simulators is strongly encouraged during the early phase of the development. However, the developers should not rely entirely on the use of simulators since sometimes, what works in the simulators may not work in real device.
Scalability to accommodate more devices. Designing IoT systems that can efficiently handle increasing numbers of devices requires proper planning to provision for future devices, which may use different communication protocols and technologies. To overcome such issue, we believe that adopting microservice architecture for IoT systems can help to overcome such challenge.
Lack of white box testing. Writing robust code requires testing before deployment. However, many IoT devices lack the capability to execute unit tests. Although developers may not be able to conduct unit tests, we believe that adopting a microservice architecture would allow testers to test each endpoint. This ensures that the app deployed on such a device will work as expected.